Bài giảng Lập trình hướng đối tượng - Chương 6: Standard Template Library
Origins of STL
Generic programming
STL components
STL installation
STL header files
STL incompatibilities

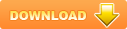
Tóm tắt nội dung Bài giảng Lập trình hướng đối tượng - Chương 6: Standard Template Library, để xem tài liệu hoàn chỉnh bạn click vào nút "TẢI VỀ" ở trên
at is very similar to a vector, except in terms of performance and memory allocation. Elements can be inserted and deleted very efficiently at either end of a deque. Deques Most other deque operations are slightly slower than the corresponding vector operations. Inserting or deleting elements in the middle of a deque is quite slow, so deques should be avoided if this kind of operation is common. Lists Unlike vectors and deques, lists are implemented as doubly-linked lists. The advantage of a list is that the time it takes to insert or delete an element is constant regardless of the element's position. Lists The disadvantage of a list is that it doesn’t fully support random access, which generic algorithms such as sort(), splice() and merge() rely on. These operations are provided as member functions of the list class instead. Lists Lists are useful when you're performing a lot of arbitrary insertions and deletions, but they're not as efficient as deques when you're inserting or deleting elements at either the start or end, and they're not as efficient as vectors when you're inserting or deleting elements at the end only. Vector functions The simplest sequential container, the vector, will be used to illustrate the functions that are common to all STL containers. Vector constructors vector()Constructs an empty vector. vector(size_type n)Constructs a vector containing n elements set to their default values. vector(size_type n, const T& value)Constructs a vector containing n elements initialised to value. vector(const T* first, const T* last)Constructs a vector containing copies of all the elements in the range [first, last). Vector copy constructor and destructor vector(const vector& aVector)Constructs a vector as a copy of aVector. ~vector()Destroys the vector and all of its elements. Vector insertion void push_back(const T& value)Adds value to the end of the vector, expanding storage to accommodate the new element if necessary. iterator insert(iterator pos, const T& value)Inserts a copy of value at pos and returns an iterator pointing to the new element's position. void insert(iterator pos, size_type n, const T& value)Inserts n copies of value at pos. void insert(iterator pos, const T *first, const T *last)Inserts copies of the elements in the range [first, last) at pos. Vector deletion void pop_back()Deletes the vector's last element. void erase(iterator pos)Deletes the element at pos. void erase(iterator first, iterator last)Deletes the elements in the range [first, last). Vector accessor functions T& back()Returns a reference to the vector's last element. T& front()Returns a reference to the vector's first element. T& operator[](int index)Returns a reference to the vector's index-th element. bool empty() constReturns true if the vector contains no elements. Vector accessor functions .. 2 size_type max_size() constReturns the maximum number of elements the vector can contain. size_type size() constReturns the number of elements the vector contains. iterator begin()Returns an iterator positioned at the vector's first element. iterator end()Returns an iterator positioned immediately after the vector's last element. Vector relational operators The == operator and the , = operators are all defined in terms of the == and & operator=(const vector& aVector)Replaces the contents of the vector with the contents of aVector. void swap(vector& aVector)Swap the contents of the vector with the contents of aVector. #include #include // Displays the contents of a vector of integers void display(const vector v); void main() { vector vec1(3, 11); vector vec2; display(vec1); Example 1 ..1 // Insert 22 at the end of the vector vec1.push_back(22); display(vec1); // Insert 33 at the beginning of the vector vec1.insert(vec1.begin(), 33); display(vec1); // Remove all elements except for the first and the last vec1.erase(vec1.begin() + 1, vec1.end() - 1); display(vec1); Example 1 ..2 // Remove the element at the end of the vector vec1.pop_back(); display(vec1); // Assign the contents of vec1 to vec2 vec2 = vec1; display(vec2); } Example 1 ..3 // Displays the contents of a vector of integers void display(const vector v) { if (v.empty()) cout #include // Displays the contents of a deque of characters void display(const deque d); void main() { deque deq(2, 'a'); display(deq); // Insert b at the beginning of the deque deq.push_front('b'); display(deq); Example 2 ..1 // Insert three c's at the end of the deque deq.insert(deq.end(), 3, 'c'); display(deq); // Remove two elements from the beginning of the deque deq.erase(deq.begin(), deq.begin() + 2); display(deq); // Remove the element at the beginning of the deque deq.pop_front(); display(deq); } Example 2 ..2 // Displays the contents of a deque of characters void display(const deque d) { if (d.empty()) cout & aList)Deletes all of the elements from aList and inserts them before position pos. void splice(iterator to, list& aList, iterator from)Deletes the element in aList at position from and inserts it at position to. void splice(iterator pos, list& aList, iterator first, iterator last)Deletes the elements from aList in the range [first, last) and inserts them at position pos. List functions .. 2 void remove(const T& value)Deletes all elements from the list that match value. void reverse()Reverses the order of elements in the list. void unique()Replaces all repeating sequences of an element with a single occurrence of that element. List functions .. 3 void sort()Sorts the list's elements into ascending order. void merge(const list aList)Assumes that the elements of both the list and aList are sorted into ascending order. Moves the elements of aList into the list while maintaining order. The operator[]() function is not available to lists, but other accessor functions are. #include #include using namespace std; // Displays the contents of a list of integers void display(const list l); int main() { list list1; list list2; // Initialise list1 to 1, 2, 3, 4, 5 for(int i = 1; i l) { list::iterator i; if (l.empty()) cout multimap set multiset Maps A map is an associative container that manages a set of ordered key/value pairs. Think of a map as an array that can be indexed by a key of any type. When a map is instantiated, a comparator function object must be specified so the map knows how to sort its key/value pairs. #include #include #include int main(void) { map > directory; directory["Kent"] = 5559283; directory["Lane"] = 5552789; directory["Olsen"] = 5553267; // etc Example 4 .. 1 // Read some names and look up their numbers string name; while (cin >> name) { if (directory.find(name) != directory.end()) cout #include using namespace std; int main() { vector nums1; nums1.insert(nums1.begin(), -999); nums1.insert(nums1.begin(), 14); nums1.insert(nums1.end(), 25); int i; for (i=0; i nums2 = nums1; nums2.insert(nums2.begin(), 32); vector nums3; nums3 = nums2; for (i=0; i #include using namespace std; int main() { int arr[] = { 100, 110, 120, 130 }; vector v(arr, arr+4); int j; cout #include using namespace std; int main() { list ilist; ilist.push_back(30); ilist.push_back(40); ilist.push_front(20); ilist.push_front(10); int size = ilist.size(); for(int j=0; j #include using namespace std; int main() { vector v; v.push_back(10); v.push_back(11); v.push_back(12); v.push_back(13); v[0] = 20; v[3] = 23; for(int j=0; j #include using namespace std; int main() { float arr[] = { 1.1, 2.2, 3.3, 4.4 }; vector v1(arr, arr+4); vector v2(4); v1.swap(v2); while( !v2.empty() ) { cout #include using namespace std; int main() { int arr1[] = { 40, 30, 20, 10 }; int arr2[] = { 15, 20, 25, 30, 35 }; list list1(arr1, arr1+4); list list2(arr2, arr2+5); list1.reverse(); list1.merge(list2); list1.unique(); int size = list1.size(); for(int j=0; j #include using namespace std; int main() { deque deq; deq.push_back(30); deq.push_back(40); deq.push_back(50); deq.push_front(20); deq.push_front(10); deq[2] = 33; for(int j=0; j #include using namespace std; class Shape { public: virtual void draw() = 0; virtual ~Shape() {}; }; class Circle : public Shape { public: void draw() { cout Container; typedef Container::iterator Iter; int main() { Container shapes; shapes.push_back(new Circle); shapes.push_back(new Square); shapes.push_back(new Triangle); for(Iter i = shapes.begin(); i != shapes.end(); i++) (*i)->draw(); for(Iter j = shapes.begin(); j != shapes.end(); j++) delete *j; return 0; } Câu 9 #include #include #include using namespace std; int main() { string names[] = {"Katie", "Robert", "Mary", "Amanda", "Marie"}; set nameSet(names, names+5); set::const_iterator iter; nameSet.insert("Jack"); nameSet.insert("Larry"); nameSet.insert("Robert"); nameSet.insert("Barry"); nameSet.erase("Mary"); cout > searchName; iter = nameSet.find(searchName); if( iter == nameSet.end() ) cout #include #include using namespace std; int main() { set city; set::iterator iter; city.insert("Trabzon"); city.insert("Adana"); city.insert("Edirne"); city.insert("Bursa"); city.insert("Ýstanbul"); city.insert("Rize"); city.insert("Antalya"); city.insert("Ýzmir"); city.insert("Hatay"); city.insert("Ankara"); city.insert("Zonguldak"); iter = city.begin(); while( iter != city.end() ) cout > lower >> upper; iter = city.lower_bound(lower); while( iter != city.upper_bound(upper) ) cout #include #include using namespace std; int main() { map city_num; city_num["Trabzon"]=61; city_num["Adana"]=01; city_num["Edirne"]=22; city_num["Bursa"]=16; city_num["Ýstanbul"]=34; city_num["Rize"]=53; city_num["Antalya"]=07; city_num["Ýzmir"]=35; city_num["Hatay"]=31; city_num["Ankara"]=06; city_num["Zonguldak"]=67; string city_name; cout > city_name; if (city_num.end()== city_num.find(city_name)) cout << city_name << " is not in the database" << endl; else cout << "Number of " << city_name << ": " << city_num[city_name]; return 0; } Hỏi và Đáp
File đính kèm:
Bài giảng Lập trình hướng đối tượng - Chương 6 Standard Template Library.ppt