Bài giảng Lập trình hướng đối tượng - Chương 1: Lớp và đối tượng
Kiểu dữ liệu trừu tượng (Abstract data type)
Lớp
Đối tượng
Ngôn ngữ UML
Các ví dụ
Các phương thức tạo/hủy đối tượng
Con trỏ this

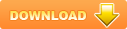
Tóm tắt nội dung Bài giảng Lập trình hướng đối tượng - Chương 1: Lớp và đối tượng, để xem tài liệu hoàn chỉnh bạn click vào nút "TẢI VỀ" ở trên
jectName) { // ... } * Ví dụ: Conversion constructor #ifndef STUDENT_H #define STUDENT_H class Student { public: // Constructor Student(char* aName = ""); private: char* name; // Student's name int numUnits;// Number of units student is enrolled in }; #endif #include "student.h“ // Constructor Student::Student(char* aName) { int length = strlen(aName) + 1; name = new char [length]; strcpy(name, aName); numUnits = 0; } #include "student.h" void enrol(Student aStudent); // ... void main(void) { enrol("Jim Shooz"); // ... } * Hủy đối tượng class Account { private: char *name; double balance; unsigned int id; //unique public: Account(); Account(const Account &a); Account(const char *person); ~Account(); } Destructor Account :: ~Account() { delete[] name; } Its name is the class name preceded by a ~ (tilde) It has no argument It is used to release dynamically allocated memory and to perform other "cleanup" activities It is executed automatically when the object goes out of scope * Ví dụ: Hủy đối tượng class Str { char *pData; int nLength; public: //constructors Str(); Str(char *s); Str(const Str &str); //accessors char* get_Data(); int get_Len(); //destructor ~Str(); }; Str :: Str() { pData = new char[1]; *pData = ‘\0’; nLength = 0; }; Str :: Str(const Str &str) { int n = str.nLength; pData = new char[n+1]; nLength = n; strcpy(pData,str.pData); }; Str :: Str(char *s) { pData = new char[strlen(s)+1]; strcpy(pData, s); nLength = strlen(s); }; * Ví dụ: Hủy đối tượng (tt) class Str { char *pData; int nLength; public: //constructors Str(); Str(char *s); Str(const Str &str); //accessors char* get_Data(); int get_Len(); //destructor ~Str(); }; char* Str :: get_Data() { return pData; }; Str :: ~Str() { delete[] pData; }; int Str :: get_Len() { return nLength; }; * Ví dụ: Hủy đối tượng (tt) class Str { char *pData; int nLength; public: //constructors Str(); Str(char *s); Str(const Str &str); //accessors char* get_Data(); int get_Len(); //destructor ~Str(); }; int main() { int x=3; Str *pStr1 = new Str(“Joe”); //initialize from char* Str *pStr2 = new Str(); //initialize with constructor } * Hình ảnh bộ nhớ int x 3 type var value Str * pStr1 Address Str * pStr2 Address char * pData address type var value int nLength strlen("joe")+1 Stack Memory Heap Memory Str object Str object char * pData address type var value int nLength 0 char '\0' type value char 'J' char 'o' char 'e' char '\0' * Các giai đoạn trong thời gian sống của một đối tượng bits * Các loại phương thức Có các loại hàm thành viên khác nhau trong khai báo của một lớp accessor int CStr :: get_length(); implementor/worker void Rectangle :: set(int, int); helper/utility void Date :: errmsg(const char* msg); constructor Account :: Account(); Account :: Account(const Account& a); Account :: Account(const char *person); destructor Account :: ~Account(); Các phương thức còn lại * Phương thức get/set * Phạm vi Chúng ta có thể sử dụng một biến hoặc một đối tượng chỉ trong một phạm vi cho phép của nó. Các phạm vi: local (or block) scope/cục bộ/khối global scope/toàn cục class scope/lớp file scope/tập tin * Phạm vi cục bộ In C++ a block of code is a section of code between { }. A local variable is in scope from it’s declaration to the end of the block { int x , y = 2; x = 1; { int x2 = 4, int y; x = x2; y = 5; } cout = 0) dollars = d; else dollars = 0; } A B A B * Phạm vi file To declare a variable with file scope, declare it outside any block or class and precede it with the keyword static. It is in scope from the declaration to the end of the file. static int myVal; void myFun(void); int main (void){ myVal = 0; myFun(); } void myFun() { myVal++; } * Thành viên dữ liệu tĩnh/không tĩnh Thành viên dữ liệu không tĩnh Mỗi một đối tượng của lớp có một bản sao giá trị sở hữu của nó Thành viên tĩnh Như là một biến toàn cục Một bản sao cho một lớp. Ví dụ như bộ đếm * Thành viên dữ liệu tĩnh class Rectangle { private: int width; int length; static int count; public: void set(int w, int l); int area(); } Rectangle r1; Rectangle r2; Rectangle r3; width length width length width length r1 r3 r2 count * Hàm thành viên tĩnh Giống như dữ liệu thành viên tĩnh, là một hàm toàn cục, tất cả các đối tượng của lớp chia sẻ hàm này Không có con trỏ this (sẽ đề cập sau) Không thể truy cập các thành viên không tĩnh của lớp Không phải là hàm thành viên hằng Một hàm thành viên có thể gọi một hàm thành viên tĩnh thuộc lớp đó một cách trực tiếp Một hàm không thành viên có thể gọi một hàm thành viên tĩnh bằng cách: ClassName::staticMemberFunctionName() or objectName.staticMemberFunctionName() * Ví dụ: Thành viên tĩnh Student class -studentCount +getStudentCount Student student1; Student student2; Student::getStudentCount(); student1.setID( 1 ); student2.setID( 2 ); class Student { private: long id; static long studentCount; public: Student() { studentCount++; } static long getStudentCount() { return studentCount; } long getID() { return id; } void setID( long inID ) { id = inID; } }; Sử dụng thành viên tĩnh khi nào ? * Ví dụ: Thành viên tĩnh (tt) * Thành viên dữ liệu hằng Ta đã biết từ khóa const dùng với các biến const int x = 50; Từ khóa const đối với thành viên dữ liệu ? Khi một thành viên dữ liệu được khai báo là const, thành viên đó sẽ giữ nguyên giá trị trong suốt thời gian sống của đối tượng chủ * Thành viên dữ liệu hằng (tt) Khởi tạo thành viên dữ liệu hằng khi nào ? Bên trong khai báo lớp? Quá sớm, ta chưa có đối tượng nào, không có chỗ để lưu giá trị Gán trị trong thân constructor? Quá muộn, không đảm bảo hằng không được truy cập trước khi nó được gán Giải pháp: danh sách khởi tạo tại constructor Danh sách khởi tạo của constructor nằm tại định nghĩa của constructor, khi dùng các hàm thành viên, danh sách khởi tạo đảm bảo chúng được khởi tạo trước khi được truy cập * Thành viên dữ liệu hằng (tt) Các hằng của các đối tượng khác nhau (thuộc cùng một lớp) không có quan hệ gì với nhau Ví dụ, một đối tượng thuộc lớp MyClass có hằng foo với giá trị 5, trong khi đó một đối tượng khác cùng thuộc lớp MyClass lại có hằng foo có giá trị 10 * Hàm thành viên hằng Khai báo return_type func_name (para_list) const; Định nghĩa return_type func_name (para_list) const { … } return_type class_name :: func_name (para_list) const { … } Không được thay đổi các thành viên dữ liệu (safe function) Không hợp lệ, nếu một hàm thành viên hằng thay đổi thành viên dữ liệu của lớp * Ví dụ: Hàm thành viên hằng class Time { private : int hrs, mins, secs ; public : void write ( ) const ; } ; void Time :: write( ) const { cout id = id; } }; * Con trỏ this (tt) Example 2 – cascading method calls class Student { private: long id; bool enrolled; public: long getID() { return id; } Student& setID( long id ) { this->id = id; return *this; } bool isEnrolled() { return enrolled; } Student& setEnrolled( bool enrolled ) { this->enrolled = enrolled; return *this; } }; void main() { Student student; student.setEnrolled( true ).setID( 1 ); } Tại sao kiểu trả về phải là tham chiếu ? * Con trỏ this (tt) Example 3 – passing your instance around #include using namespace std; // constants const int MAX_STRING_LENGTH = 256; const int MAX_STUDENTS = 20; // class Student interface class Student { private: static long studentCount; static Student* studentList[ MAX_STUDENTS ]; char name[ MAX_STRING_LENGTH ]; bool enrolled; long id; public: Student( char*, bool ); const char* getName(); void setName( char* ); bool isEnrolled(); void setEnrolled( bool ); long getID(); static Student* getStudentFromID( long id ); static long getStudentCount(); }; // class Student implmentation Student::Student( char* name, bool enrolled ) { setName( name ); setEnrolled( enrolled ); id = studentCount; studentList[ Student::studentCount ] = this; studentCount++; } const char* Student::getName() { return name; } void Student::setName( char* name ) { strcpy( this->name, name ); } bool Student::isEnrolled() { return enrolled; } void Student::setEnrolled( bool enrolled ) { this->enrolled = enrolled; } * Con trỏ this (tt) Example 3 – passing your instance around – cont’d long Student::getID() { return id; } // class Student static method implmentation Student* Student::getStudentFromID( long id ) { if ( ( id = 0 ) ) { return studentList[ id ]; } else { return 0; } } long Student::getStudentCount() { return studentCount; } // class Student static member initialization long Student::studentCount = 0; Student* Student::studentList[ MAX_STUDENTS ]; // main routine to demonstrate Student void main() { // get students do { char name[ MAX_STRING_LENGTH ]; bool enrolled; cout > name; if ( stricmp( name, "end" ) == 0 ) break; cout > enrolled; new Student( name, enrolled ); } while( true ); * Con trỏ this (tt) Example 3 – passing your instance around – cont’d // print list of students for( int i = 0; i getName() getID() isEnrolled() using namespace std; class Student { int dept; public: Student(int d) {dept=d; } bool sameDept(const Student &st) { if (dept==st.dept) return true; else return false; } }; int main() { Student st1(5), st2(6); if (st1.sameDept(st2)==true) cout using namespace std; class Teacher { int dept; public: Teacher(int d) { dept=d; } }; class Student { int dept; public: Student(int d) { dept=d; } bool TsameDept(const Teacher &tch) { if (dept==tch.dept) return true; else return false; } }; int main() { Student st(5); Teacher tch(5); if (st.TsameDept(tch)== true) cout Using namespace std; class Point { public: Point() { cout << "Point constructor\n"; } ~Point() { cout << "Point destructor\n"; } }; const int MAXVERTICES = 3; class Figure { public: Figure() { cout << "Figure constructor\n"; } ~Figure() { cout << "Figure destructor\n"; } private: Point vertex[MAXVERTICES]; // //array of vertices Point center; }; int main() { Figure F; return 0; } Kết quả: ? * * Hỏi và Đáp *
File đính kèm:
Bài giảng Lập trình hướng đối tượng - Chương 1 Lớp và đối tượng.ppt